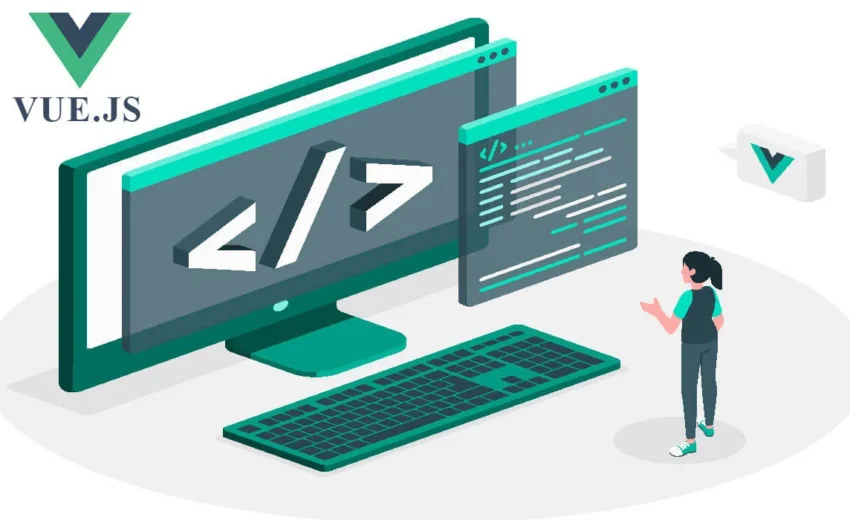
Search Engine Optimization (SEO) is crucial for ensuring your Vue.js 3 application is discoverable by users across different languages. In this guide, we’ll explore how to implement multilingual SEO using Vue.js 3 Composition API, along with the @vueuse/head
library for managing head metadata.
Prerequisites
Before diving into the implementation, ensure you have the necessary packages installed and your project structure set up:
1. Install @vueuse/head
:
npm install @vueuse/head
2. Create a language file (ar.json
) to store translations for both Arabic and English.
// ar.json
{
“title”: “عنوان1”,
“description”: “وصف1”
}
3. Set up your settingStore.js
file:
// settingStore.js
import { defineStore } from ‘pinia’;
export const useSettingsStore = defineStore(() => {
const lang = ref(document.documentElement.lang);
return {
lang,
};
});
Configuring Head Metadata
Now, let’s integrate the @vueuse/head
library to dynamically update metadata in the head
of your Vue.js application.
1. Configure app.js
:
// app.js
import { createApp } from ‘vue’;
import { createHead } from ‘@vueuse/head’;
import App from ‘./App.vue’;
const app = createApp(App);
const head = createHead();
app.use(head);
2. Implement SEO in home.vue
:
<!– home.vue –>
<template>
<div>
<!– Your component content here –>
</div>
</template>
<script setup>
import { useSettingsStore } from ‘../stores/settingsStore’;
import { Head } from ‘@vueuse/head’;
import { ref, computed, watch } from ‘vue’;
import { useI18n } from ‘vue-i18n’;
const { t, locale } = useI18n();
const store = useSettingsStore();
const keywords = {
“en”: [“key1”, “key2”, “key3”],
“ar”: [“key3”, “key2”, “key1″]
};
const localeKeywords = ref(keywords[store.lang] || []);
const keywordsString = computed(() => localeKeywords.value.join(‘, ‘));
watch(
() => store.lang,
(newLocale) => {
localeKeywords.value = keywords[newLocale] || [];
}
);
</script>
<Head>
<title>{{ t(‘Paseetah – Real Estate Data Platform’) }}</title>
<meta name=”description” :content=”t(‘Paseetah is a Real Estate Data Company offering information, tools, and insights and analyses to help make better real estate decisions.’)”>
<meta name=”keywords” :content=”keywordsString”>
</Head>
In this example, we’ve utilized the Head
component from @vueuse/head
to dynamically update the <head>
section of your HTML document based on the selected language.
Conclusion
Implementing multilingual SEO in Vue.js 3 Composition API involves managing dynamic metadata and translations. By using the @vueuse/head
library and the Composition API features, you can create a flexible and SEO-friendly application that caters to users in different languages. Keep in mind that regularly updating and maintaining your SEO strategy is essential for optimal search engine visibility.